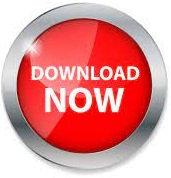
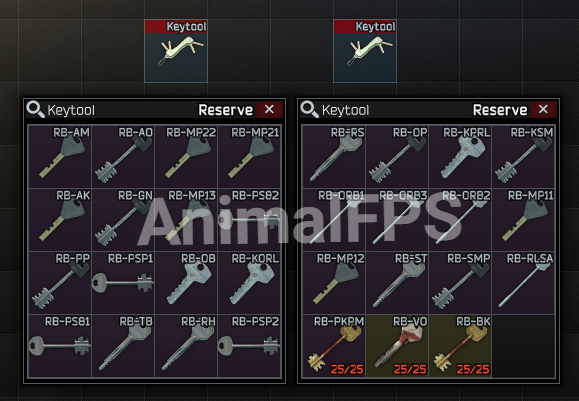
The attack aims at getting the worst-case algorithmic behavior (O(n) instead of amortized O(1), see Algorithmic Complexity for the details) when the data is fed into the table. Algorithmic complexity attacksĪll hash tables are vulnerable to a particular class of denial of service attacks, in which the attacker carefully pre-computes a set of different keys that are going to be hashed in the same bucket of a hash table (or even have the very same hash value). You must not rely on the fact that qHash() will give the same results (for the same inputs) across different Qt versions. Note that the implementation of the qHash() overloads offered by Qt may change at any time. In the example above, we've relied on Qt's global qHash(const QString &, uint) to give us a hash value for the employee's name, and XOR'ed this with the day they were born to help produce unique hashes for people with the same name. Inline uint qHash( const Employee &key, uint seed) Inline bool operator =( const Employee &e1, const Employee &e2) #ifndef EMPLOYEE_H #define EMPLOYEE_H class EmployeeĮmployee( const QString &name, QDate dateOfBirth) If you want to use other types as the key, make sure that you provide operator=() and a qHash() implementation. Many other Qt classes also declare a qHash overload for their type please refer to the documentation of each class. For all of these, the header defines a qHash() function that computes an adequate hash value. Here's a partial list of the C++ and Qt types that can serve as keys in a QHash: any integer type (char, unsigned long, etc.), any pointer type, QChar, QString, and QByteArray. If both a one-argument and a two-arguments overload are defined for a key type, the latter is used by QHash (note that you can simply define a two-arguments version, and use a default value for the seed parameter). This seed is provided by QHash in order to prevent a family of algorithmic complexity attacks. The two-arguments overloads take an unsigned integer that should be used to seed the calculation of the hash function. However, to obtain good performance, the qHash() function should attempt to return different hash values for different keys to the largest extent possible.įor a key type K, the qHash function must have one of these signatures: In other words, if e1 = e2, then qHash(e1) = qHash(e2) must hold as well. It can use any algorithm imaginable, as long as it always returns the same value if given the same argument. The qHash() function computes a numeric value based on a key. The qHash() hashing functionĪ QHash's key type has additional requirements other than being an assignable data type: it must provide operator=(), and there must also be a qHash() function in the type's namespace that returns a hash value for an argument of the key's type. You cannot, for example, store a QWidget as a value instead, store a QWidget *. QHash's key and value data types must be assignable data types.
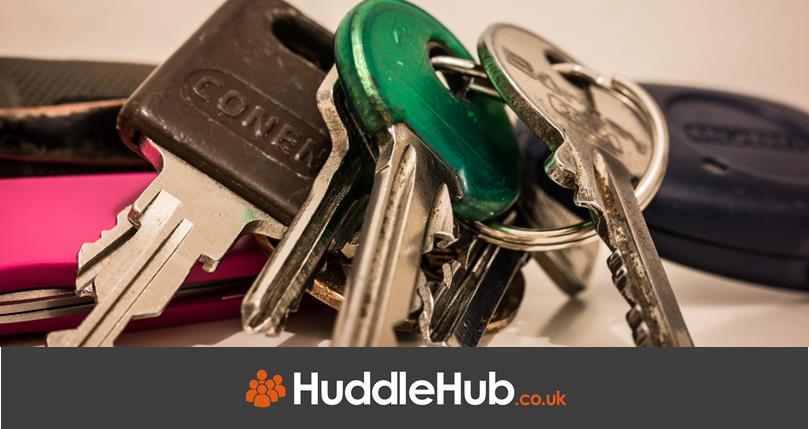
In addition, you can clear the entire hash using clear(). Another way is to use QMutableHashIterator::remove(). One way is to call remove() this will remove any item with the given key. Items can be removed from the hash in several ways. Operator>(QDataStream & in, QHash & hash) QHashRangeCommutative(InputIterator first, InputIterator last, uint seed = 0) QHashRange(InputIterator first, InputIterator last, uint seed = 0) QHashBits(const void * p, size_t len, uint seed = 0) QHash(const QStringRef & key, uint seed = 0) QHash(const QString & key, uint seed = 0) QHash(const QByteArray & key, uint seed = 0) QHash(const QVersionNumber & key, uint seed = 0) QHash(const std::pair & key, uint seed = 0) QHash(QSslEllipticCurve curve, uint seed) QHash(const QDateTime & key, uint seed = 0) QHash(const QBitArray & key, uint seed = 0) QHash(const QOcspResponse & response, uint seed) QHash(const QSslDiffieHellmanParameters & dhparam, uint seed) Value(const Key & key, const T & defaultValue) const Key(const T & value, const Key & defaultKey) const QHash(InputIterator begin, InputIterator end)
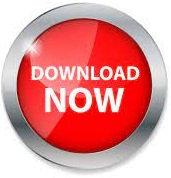